09. The DOM
The DOM Header
The DOM
Understanding HTML hierarchy is important because child elements can inherit behavior and styling from their parent element.
ND001 C01 L01 06 Intro To The DOM
Document type declaration
Note: If you’d like to inspect the HTML of a website as Daniel does in the video, you can use Chrome’s Developer Tools to do so.
Now that you’ve learned about some of the most common HTML elements, it’s time to learn how to set up an HTML file. HTML files require certain elements to set up the document properly. You can let web browsers know that you are using HTML by starting your document with a document type declaration. The declaration looks like this:
<!DOCTYPE html>
This declaration is an instruction, and it must be the first line of code in your HTML document. It tells the browser what type of document to expect, along with what version of HTML is being used in the document. For now, the browser will correctly assume that the html in <!DOCTYPE html>
is referring to HTML5, as it is the current standard.
In the future, however, a new standard will override HTML5. To make sure your document is forever interpreted correctly, always include <!DOCTYPE html>
at the very beginning of your HTML documents.
The Head
The Head
So far you’ve done two things to set up the file properly:
- Declared to the browser that your code is HTML with
<!DOCTYPE html>
- Added the HTML element (
<html>
) that will contain the rest of your code.
Remember the <body>
tag? The <head>
element is part of this HTML metaphor. It goes above our <body>
element.
Metadata
The <head>
element contains the metadata for a web page.
Metadata is information about the page that isn’t displayed directly on the web page.
Unlike the information inside of the <body>
tag, the metadata in the head is information about the page itself.
Title
A browser’s tab displays the title specified in the <title
> tag. The <title>
tag is always inside of the <head>
.
<!DOCTYPE html>
<html>
<head>
<title>My Coding Journal</title>
</head>
</html>
If we were to open a file containing the HTML code in the example above, the browser would display the words “My Coding Journal” in the title bar (or in the tab’s title).
The Head Graphic
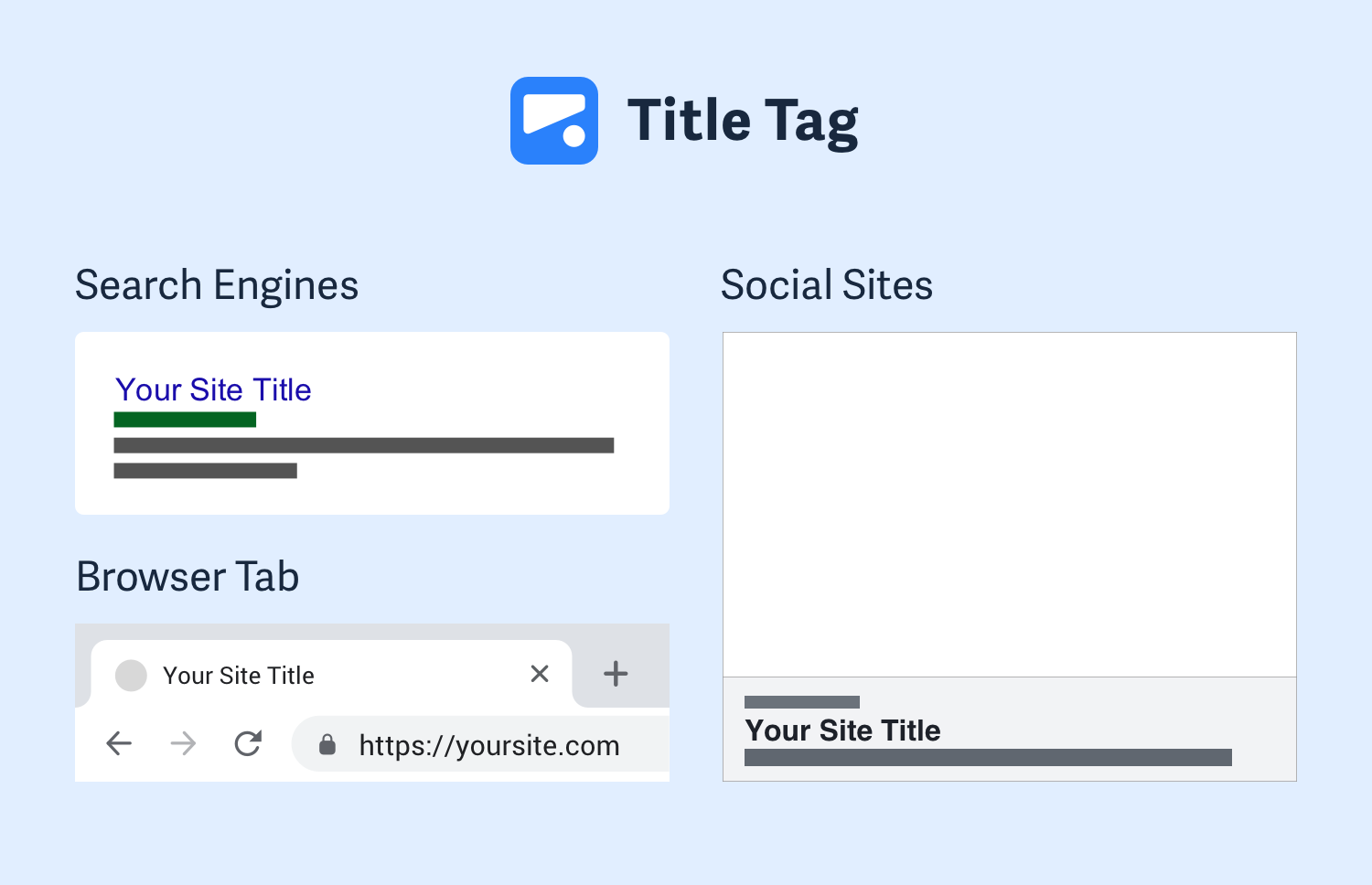
An example of titles
The Body
The Body
<body></body>
Once the file has a body, many different types of content – including text, images, and buttons – can be added to the body.
Hierarchy
Hierarchy
HTML is organized as a collection of family tree relationships. As you saw in the last exercise, we placed <p>
tags within <body>
tags. When an element is contained inside another element, it is considered the child of that element. The child element is said to be nested inside of the parent element.
<body>
<p>This paragraph is a child of the body</p>
</body>
In the example above, the <p>
element is nested inside the <body>
element. The <p>
element is considered a child of the <body>
element, and the <body>
element is considered the parent. You can also see that we’ve added two spaces of indentation (using the space bar) for better readability.
Since there can be multiple levels of nesting, this analogy can be extended to grandchildren, great-grandchildren, and beyond. The relationship between elements and their ancestor and descendent elements is known as hierarchy.
Let’s consider a more complicated example that uses some new tags:
<body>
<div>
<h1>Sibling to p, but also grandchild of body</h1>
<p>Sibling to h1, but also grandchild of body</p>
</div>
</body>
In this example, the <body>
element is the parent of the <div>
element. Both the <h1>
and <p>
elements are children of the <div>
element. Because the <h1>
and <p>
elements are at the same level, they are considered siblings and are both grandchildren of the <body>
element.
Again, understanding HTML hierarchy is important because child elements can inherit behavior and styling from their parent element. You’ll learn more about webpage hierarchy when you start digging into CSS.
Semantic Elements
Semantic Elements
Structure elements allow you to organize the main parts of your page. They usually contain other HTML elements.
Here’s what a typical webpage could include:
<header>
as the first element of the page, that can include the logo and the tagline.<nav>
as a list of links that go to the different pages of the website.<h1>
as the title of the page.<article>
as the main content of the page, like a blog post.<footer>
as the last element of the page, located at the bottom.
Semantic Elements Graphic
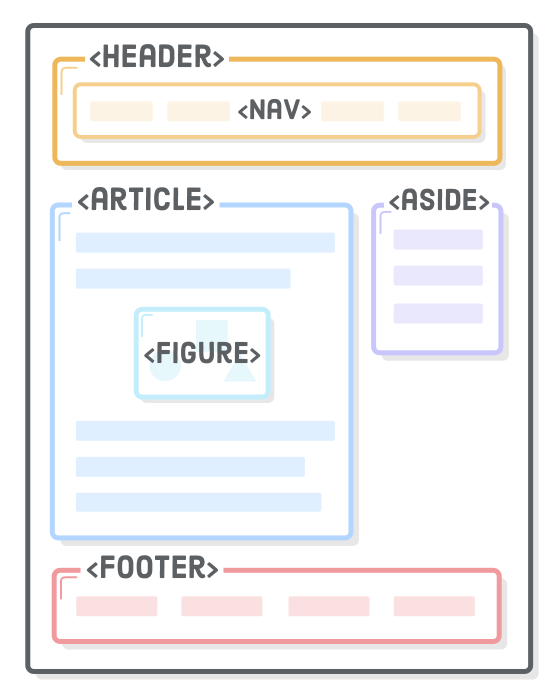
An example of the structure of semantic elements